Misaki escreveuFor example, you can replace your skeleton's mipmaps via the following steps:
1.Enable Advanced - Read/Write
and Generate Mip Maps
in your skeleton's texture Inspector.
2.Add textures to replace mipmaps to your Unity project, and enable Advanced - Read/Write
of them.
3.Create a C# script with the following code:
using UnityEditor;
using UnityEngine;
public class MipMapReplacing : AssetPostprocessor
{
private void OnPostprocessTexture( Texture2D texture )
{
// Loads textures to be set as mipmaps
var tex1 = AssetDatabase.LoadAssetAtPath<Texture2D>( "Your texture's path1.png" );
var tex2 = AssetDatabase.LoadAssetAtPath<Texture2D>( "Your texture's path2.png" );
// Set mipmap texture. The second argument is the MipMap level
texture.SetPixels( tex1.GetPixels( 0 ), 1 );
texture.SetPixels( tex2.GetPixels( 0 ), 2 );
texture.Apply( false );
}
}
- Right click your skeleton's texture and click
Reimport
in the menu.
If you try this and find line artifacts appear around the mipmap texture, please check the forum post I mentioned first.
Okay, this makes much more sense to me! 8)
Though, I am unfamiliar with the OnPostprocessTexture() method. Once I create this script, where do I call it? Would I call this method in Start() for every single character?
May I please see an example of how you use it once the script is created? Thank you very much, Misaki! 🙂
Harald escreveu
Regarding providing your own pre-authored mipmaps and loading dds files:
Texture access is provided by Unity, not by the spine-unity runtime, so if you should find any thirdparty asset which allows loading dds files to textures including mipmaps, these textures will then likely work with the spine-unity runtime as well.
Thanks for the reply, dude! 🙂
Though, I'm a bit confused by your response. What third party asset are you referring to?
Unity natively supports .DDS textures.
For example:
If I have a plane/quad in Unity, and I create a material with the following .DDS texture. Unity is automatically smart enough to know use the mipmaps provided in the .DDS file instead of creating its own.
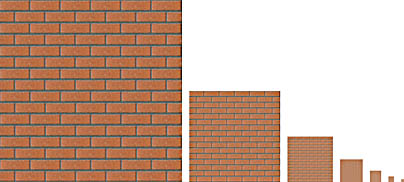
That being said, I understand how mipmaps work in Unity. What I don't understand is how the Spine atlas uses the material. Does it work the same way any other model + material works? In other words, if I simply drag a .DDS file into the material like the example below, is anything unique happening behind the scenes, or will it allow Unity to use the mipmaps in the .DDS texture file?

I really feel like there should be any kind of documentation on this.
It is not simply a "Unity thing", because Unity supports .DDS files and custom mipmaps easily. 🙁